Vue Js Ternary Operator Example:The Vue.js ternary operator is a concise way to handle conditional rendering in Vue.js templates. It works by evaluating a Boolean expression, and rendering one of two possible outcomes based on its result. The ternary operator has the syntax of condition ? trueOutcome : falseOutcome
. If the condition is true, the trueOutcome is rendered, otherwise, the falseOutcome is rendered. This feature can be useful for displaying or hiding elements, setting CSS classes or styles, and even rendering dynamic content. Overall, the Vue.js ternary operator is a handy tool for creating responsive and dynamic user interfaces in Vue.js applications
How do you use the ternary operator in Vue js to conditionally render content based on a boolean value?
This code block is a Vue.js application that demonstrates the use of a ternary operator to conditionally render content based on the value of a data property.
In this example, the showMessage
data property is set to true
by default. The ternary operator is used to determine whether to display the “Hello, world!” message based on the value of showMessage
. If showMessage
is true, the message will be displayed; otherwise, an empty string will be rendered.
The button element includes an event listener that toggles the value of showMessage
when clicked. The text of the button changes dynamically based on the value of showMessage
, using another ternary operator. If showMessage
is true, the button will display “Hide Message”; if it’s false, it will display “Show Message”.
This code uses the Vue.js framework to create a reactive UI, which means that any changes to the data will trigger an update to the rendered content. In this case, clicking the button will toggle the value of showMessage
, which will in turn update both the message displayed and the text of the button.
Vue Js Ternary Operator Example
<div id="app">
<h2>{{ showMessage ? 'Hello, world!' : '' }}</h2>
<button @click="showMessage = !showMessage ">{{ showMessage ? 'Hide Message' : 'Show Message' }}</button>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
showMessage: true
}
},
})
app.mount('#app')
</script>
Output of Vue Js Ternary Operator
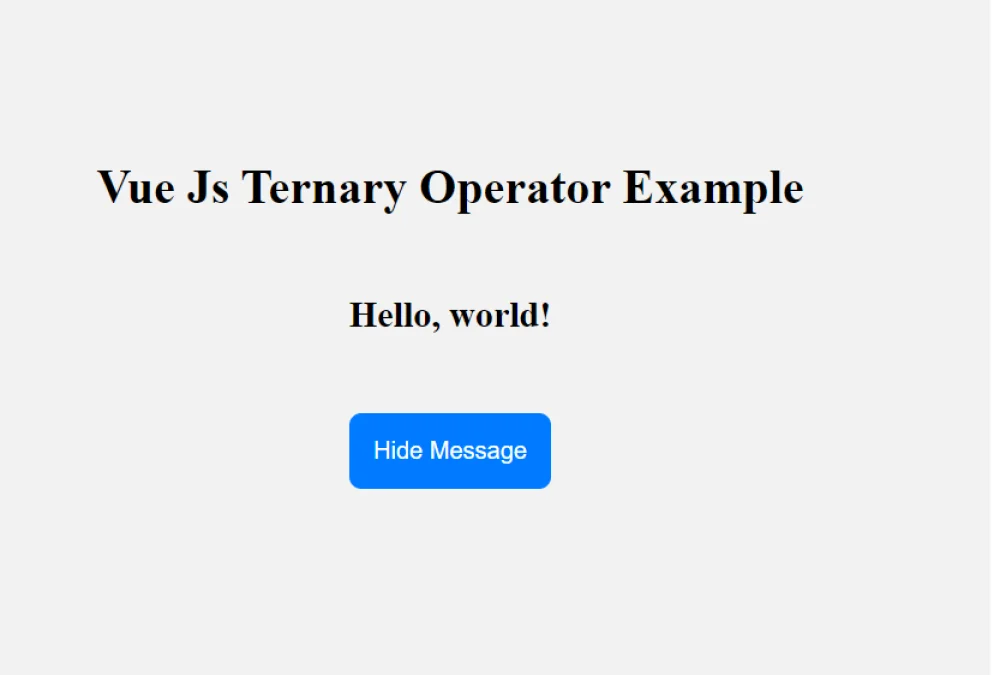